The Transformer architecture, introduced in the paper "Attention Is All You Need" by Vaswani et al. (2017), revolutionized sequence-to-sequence tasks, particularly in Natural Language Processing (NLP). Unlike previous architectures like Recurrent Neural Networks (RNNs) or Long Short-Term Memory networks (LSTMs) that process sequences token by token, Transformers process entire sequences simultaneously using attention mechanisms. This allows for significant parallelization and has led to breakthroughs in machine translation, text summarization, question answering, and the development of large language models (LLMs).
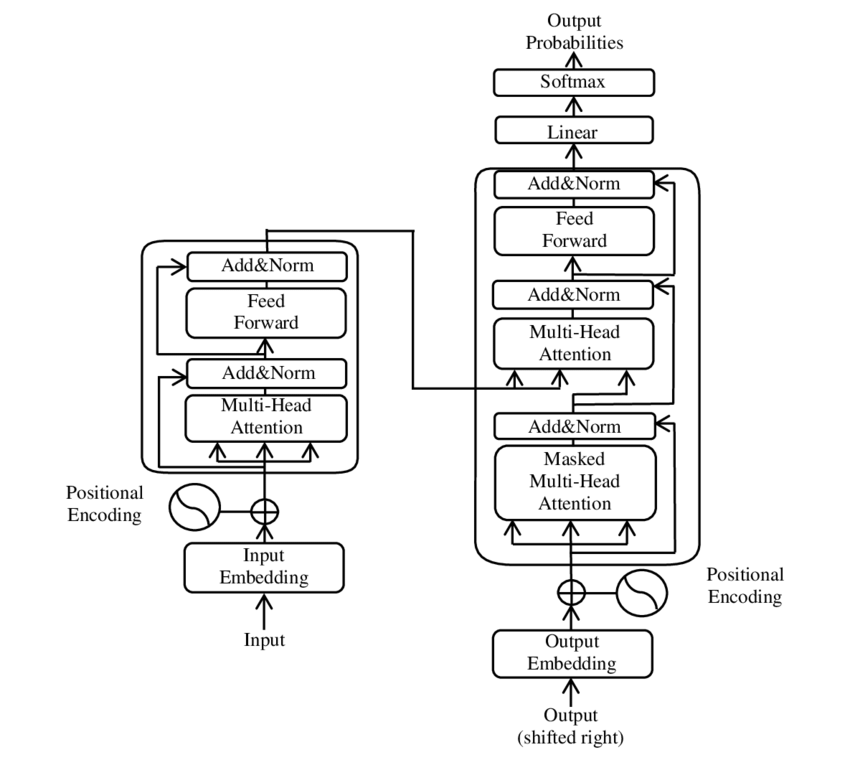
The Transformer Architecture: Encoder-Decoder structure with attention mechanisms
The core innovation of the Transformer is its reliance on self-attention mechanisms to compute representations of its input and output without using sequence-aligned RNNs or convolution.
For related content:
- LLM-specific applications and extensions:
api/content/modern_ai/llms/transformer_architecture.py
- Detailed exploration of attention mechanisms:
api/content/modern_ai/llms/attention_mechanisms.py